SQL Query using min and max aggregate function
You may want to find Customer that only has only bought one particular product in the Sales table
You will know the Product_ID is the in the Products table, in this example we use ProductID=10
In this example for each client you will find the lowest value Product_ID and the highest value Product_ID, then filters rows to those where Product_ID is the same as the variable.
--Written by Rishka Booran-Johnson
-- Get Customers with only one product
CREATE TABLE #Customers (
customer_ID int,
firstname varchar(30),
lastname varchar(30)
)
CREATE TABLE #Sales (
Transaction_ID int,
Customer_ID int,
Product_ID int,
Sale_date datetime
)
CREATE TABLE #Products (
Product_ID int,
Product_name varchar(30),
Product_description varchar(50)
)
INSERT #customers SELECT 1, 'john', 'smith'
INSERT #customers SELECT 2, 'sally', 'johnson'
INSERT #customers SELECT 3, 'joe', 'bloggs'
INSERT #Products SELECT 1, 'bicycles', ''
INSERT #Products SELECT 2, 'trains', ''
INSERT #Products SELECT 3, 'dolls', ''
INSERT #Sales SELECT 1, 1, 2, '2009-06-01'
INSERT #Sales SELECT 2, 1, 1, '2009-06-08'
INSERT #Sales SELECT 2, 1, 1, '2009-06-07'
INSERT #Sales SELECT 3, 2, 2, '2009-06-12'
INSERT #Sales SELECT 4, 2, 2, '2009-06-05'
INSERT #Sales SELECT 5, 3, 3, '2009-06-06'
INSERT #Sales SELECT 6, 3, 2, '2009-06-01'
INSERT #Sales SELECT 7, 3, 1, '2009-06-03'
declare
@vproduct_ID int
set @vproduct_ID = 2; -- this would be your input variable for trains
SELECT s.customer_ID, c.firstname, c.lastname, MIN(s.product_ID), MAX(s.product_ID)
FROM #sales s, #customers c
WHERE s.customer_ID = s.customer_ID
AND s.customer_ID = c.customer_ID
GROUP BY s.customer_ID, c.firstname, c.lastname
HAVING MIN(s.product_ID) = @vproduct_ID and MAX(s.product_ID) = @vproduct_ID
DROP TABLE #Customers
DROP TABLE #Sales
DROP TABLE #Products
Friday, June 26, 2009
Wednesday, June 24, 2009
How to add Adsense in blog
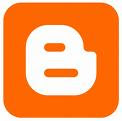
When setting up this blog, I tried to add the Adsense account gadget that Google provides, for the side bar. When selecting this I got an error of "An internal error occurred. Please try again."
What will fix this problem is :
Log into your Adsense account
Select My Account then account access.
once there you will see a section headed Hosts with access
you will see that blogger is listed but you need to click "grant access", then select it, which should change it to "disable access" !
Hope this helps those that have the same problem.
How to check database growth
Sometimes you’ll want to know how much and how fast your database has been growing. Usually you will have a document that tells you the size information on all the databases, but this is not always the case in the real world. Considering that you perform regular backups, the following script can give you a estimated idea of growth over time of your database.
select
BackupDate = convert(varchar(10),backup_start_date, 111),
SizeInGigs=floor( backup_size/1024000000)
from msdb..backupset
where
database_name = ‘DatabaseName’
and type = ‘d’
order by
backup_start_date desc
Furthermore the Backup type can be used for the following types as well , making it easier to track other types of objects:
- D = Database
- I = Differential database
- L = Log
- F = File or filegroup
- G =Differential file
- P = Partial
- Q = Differential partial
Tuesday, June 16, 2009
Keywords for your website
When looking to promote your site you want to increase traffic to your website, which is when you need good SEO (Search Engine Optimization) and is the latest buzz word around.
One of the key factors in SEO are good keywords, a keyword is what a users use to conduct a search in a search engine, such as Google, Yahoo, MSN, etc. A keyword can consist of a single word, such as “cars” or an entire phrase, like “cars for sale” or even “cars for sale in Australia”. Over time you will find out the common keyword selections website users use to find your site and you can use these keywords to try to rank your website highly in search engine results pages.
A keyword tool I use is : https://adwords.google.com/select/KeywordToolExternal
One of the key factors in SEO are good keywords, a keyword is what a users use to conduct a search in a search engine, such as Google, Yahoo, MSN, etc. A keyword can consist of a single word, such as “cars” or an entire phrase, like “cars for sale” or even “cars for sale in Australia”. Over time you will find out the common keyword selections website users use to find your site and you can use these keywords to try to rank your website highly in search engine results pages.
A keyword tool I use is : https://adwords.google.com/select/KeywordToolExternal
Saturday, June 13, 2009
RRN function in DB2
While doing some ETL I have just learned a new command in DB2 called RRN, which stands for relative record number. It has probably been used a long time, but had the rownum familiarity from Oracle.
Here is an example:
Return the relative record number and employee name from table EMPLOYEE for those employees in department 10.
SELECT RRN(EMPLOYEE), LASTNAME
FROM EMPLOYEE
WHERE DEPTNO = 10
Here is an example:
Return the relative record number and employee name from table EMPLOYEE for those employees in department 10.
SELECT RRN(EMPLOYEE), LASTNAME
FROM EMPLOYEE
WHERE DEPTNO = 10
Subscribe to:
Posts (Atom)